Master JavaScript Promises with Neutron Insights
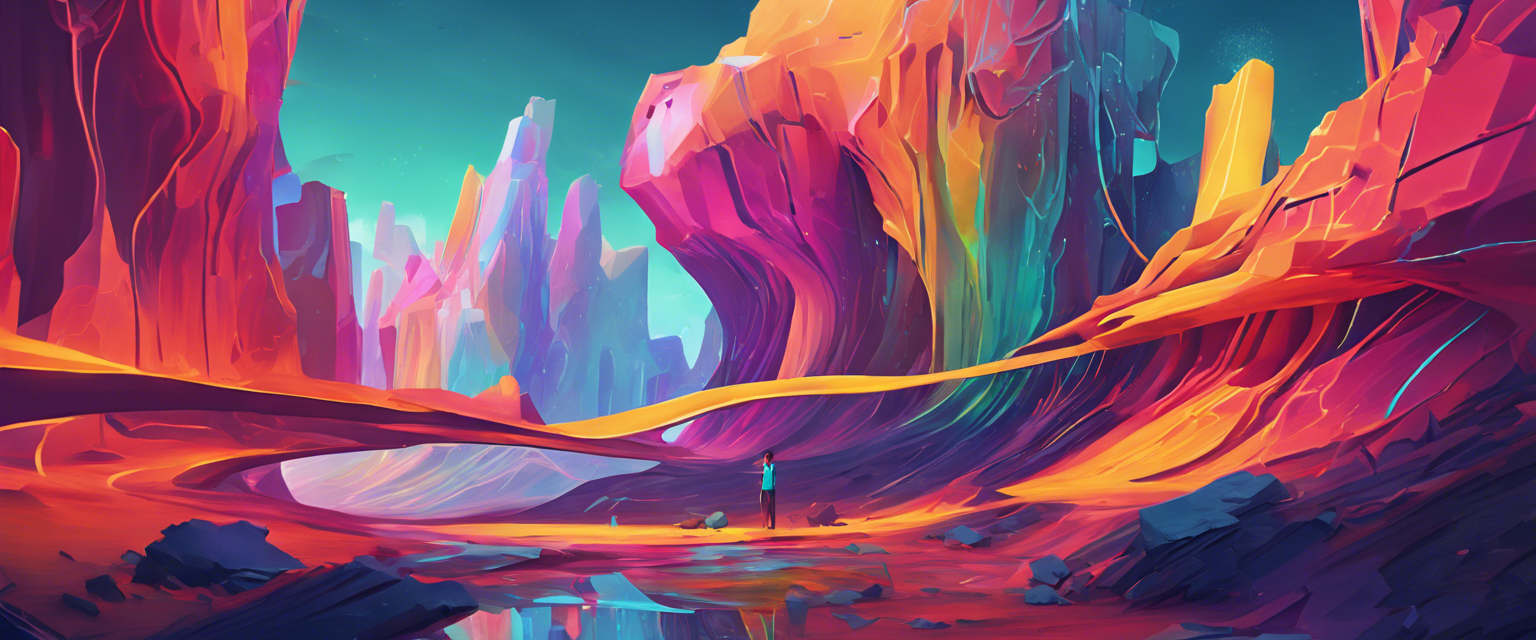
Unpacking Neutron: What You Need to Know About Its Promises and Performance
Welcome to the future of web development! Today, we’re diving into the intricate world of JavaScript promises, focusing on a fascinating piece of code that showcases how asynchronous operations can be managed seamlessly. If you’ve ever been confused about how to handle loading states or manage dependencies in your web applications, you’re in for a treat. Buckle up as we dissect the magic behind window.$neutronWindowPromises
.
What Are Promises, Anyway?
Before we delve deeper, let’s clarify what promises are in the context of JavaScript.
- Asynchronous Operations: Promises are objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value.
- State Management: They help manage multiple operations that may not complete in the order they were started.
The Code Breakdown
Let’s take a closer look at the provided code snippet. It’s packed with features that make it a powerhouse for managing promises:
- Cohesion and Tagular Promises:
window.$neutronWindowPromises['cohesion'] = new Promise((resolve) => { var value = undefined; Object.defineProperty(window, 'cohesion', { get: function () { return value; }, set: function (newValue) { value = newValue; if (value) resolve(); } }); });
Here, the cohesion
promise resolves when a value is set, offering a neat way of synchronizing operations. This pattern is replicated for tagular
and _Cohesion
, allowing multiple dependencies to be managed elegantly.
UUID Generation at Its Best
The code also includes a UUID generation function using the crypto
API for secure random values. This is a game changer for developers needing unique identifiers in their applications.
- Why UUID?
- Uniqueness: Guarantees that IDs won’t clash.
- Security: Uses cryptographic strength to ensure randomness.
Neutron’s Wait Function: A Game-Changer!
The window.neutronWaitForService
function is particularly noteworthy. It provides a mechanism to wait for a specific service to be defined before executing further code:
- Retry Logic:
- It attempts to resolve the promise for a maximum of 30 attempts, each spaced 500 milliseconds apart.
- This ensures that your application doesn’t crash waiting for a service that may take time to load.
Why Should You Care?
So, why should developers pay attention to this? Here’s the scoop:
- Simplicity: The approach makes handling asynchronous operations straightforward and clean.
- Robustness: Ensures that your web application can gracefully handle service dependencies without breaking.
- Performance Monitoring: Integrated features like BOOMR help monitor performance, making it easier to optimize your code.
Key Takeaways
- Clean State Management: Promises in this setup ensure that developers can focus on functionality without worrying about the timing of operations.
- Error Monitoring: With integrated error handling and performance monitoring, you can keep your app running smoothly.
As we continue to navigate the exciting landscape of web development, understanding and leveraging tools like Neutron’s promise handling will be crucial. It’s not just about writing code; it’s about writing smart, efficient code that enhances user experience and performance. Embrace the future and make your applications as sleek and responsive as possible!