Understanding JavaScript Promises for Developers
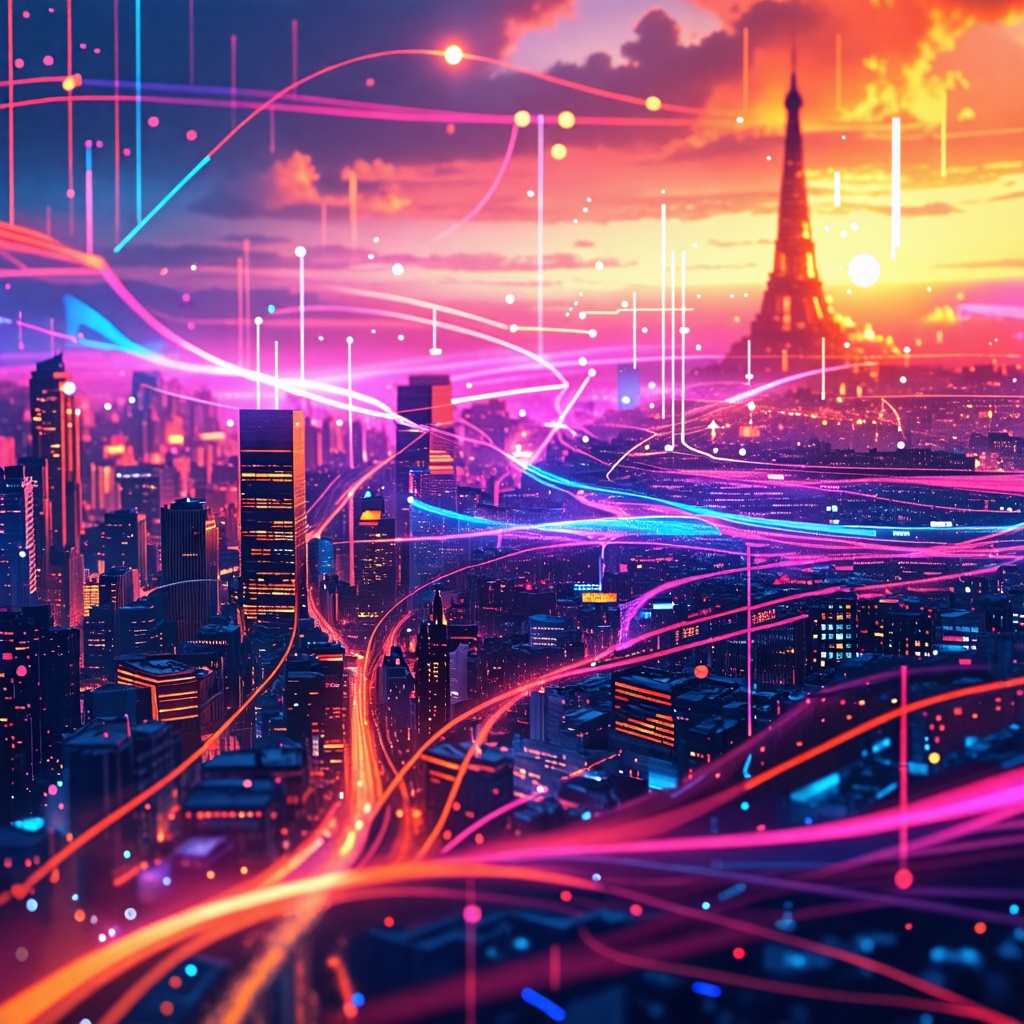
Unpacking the Complex World of JavaScript Promises: What You Need to Know!
As the Chief Editor of Mindburst.ai and an expert in AI news and product reviews, I find myself diving into the intricacies of JavaScript promises more often than I’d like to admit. If you’re a developer, you might have encountered code snippets that look like the one we’re unpacking today. What does it all mean? Let’s break it down and add some clarity to this techy puzzle!
What Are Promises, Anyway?
In JavaScript, a Promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. But why should you care? Well, understanding promises is essential if you want to write clean, efficient, and non-blocking code. For a deeper dive, check out Understanding JavaScript Promises.
Key Features of Promises:
- Pending: The initial state, neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully.
- Rejected: The operation failed.
Dissecting the Code Snippet
Let’s look at the code provided. It’s a juicy mix of promises, property definitions, and service wait functions. Here’s a breakdown:
Defining Promises
window.$neutronWindowPromises = {};
window.$neutronWindowPromises['cohesion'] = new Promise((resolve) => {
var value = undefined;
Object.defineProperty(window, 'cohesion', {
get: function () { return value; },
set: function (newValue) {
value = newValue;
if (value) resolve();
}
});
});
- This segment creates a new promise for a property called
cohesion
. The promise resolves whencohesion
is set to a truthy value. Clever, right? For a practical guide on managing asynchronous code, you might want to explore JavaScript with Promises: Managing Asynchronous Code.
Handling Multiple Promises
The snippet also defines promises for tagular
and _Cohesion
in a similar manner. These promises create a neat way to handle multiple asynchronous operations concurrently.
The Magic of neutronWaitForService
window.neutronWaitForService = (serviceName, funcName, resolve, reject) => {
var MAX_COUNT = 30, WAIT_TIME = 500;
...
};
- This function waits for a service to be defined or for a specific function to be available within a service. If the service isn't available, it retries up to a maximum count with a delay. This is a great way to ensure your application doesn’t crash due to undefined services! If you’re looking to master asynchronous JavaScript, consider Mastering Asynchronous JavaScript with Promises and Async/Await.
Why Is This Important for Developers?
- Error Handling: Promises allow for better error handling in asynchronous operations.
- Clean Code: They make your code easier to read and maintain.
- Concurrency: You can handle multiple operations simultaneously without blocking the main thread.
For those keen on advancing their JavaScript skills, 120 Advanced JavaScript Interview Questions is a fantastic resource.
Wrapping It Up: The Power of Promises
Understanding JavaScript promises is not just a trend; it's a necessity for modern web development. Whether you're defining properties dynamically or waiting for services to be available, mastering promises can significantly enhance your coding toolkit. You may also want to check out JavaScript Async: Events, Callbacks, Promises and Async Await for further insights.
So, the next time you stumble upon a promise or a complex code snippet, remember: it’s not just code; it’s a powerful tool that can help you build a better web experience. Happy coding! And if you're interested in a more practical approach, Building JavaScript A+ Promises in 10 steps! has got you covered.